Understanding JWT
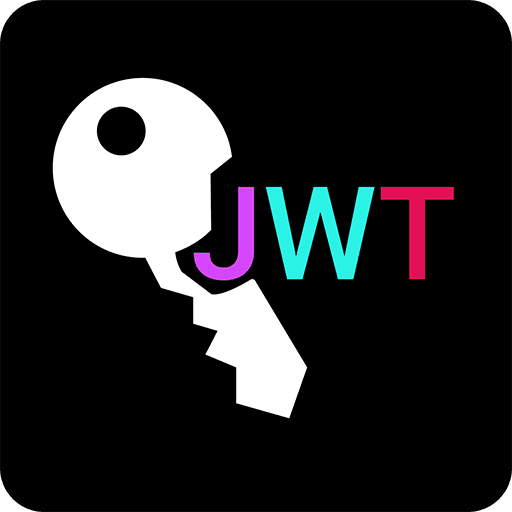
JSON Web Tokens (JWT) are a critical component in modern web security frameworks, offering a compact and secure mechanism for transmitting information between parties as a JSON object. Initially introduced to JWTs during my studies at UTD, my true depth of understanding came from hands-on application during my tenure at iServiceWeb. This blog delves into the basics of JWT, outlines its advantages in authentication, and demonstrates how embedding user-specific data such as customer IDs or emails can streamline operations and enhance security in your applications. The best way to grasp a technology is by diving into projects that utilize it, so here's a comprehensive explanation of JWT to help you start implementing it in your own projects.
What is a JSON Web Token (JWT)?
JWT is a URL-safe method of representing claims securely between two parties. The token itself is composed of three parts: a header, a payload, and a signature. Each part serves a distinct purpose:
- Header: Contains metadata about the type of token and the cryptographic algorithms used to secure it.
- Payload: Encodes the claims intended to be transmitted, along with any additional data required.
- Signature: Validates the token to ensure that the message wasn't changed along the way.
Using JWT for Authentication
JWTs are extensively used in authentication processes. Hereβs a simple example of how a JWT might be generated and validated in a NodeJS application using the Express framework:
const express = require('express');
const jwt = require('jsonwebtoken');
require('dotenv').config();
const app = express();
app.use(express.json());
const accessTokenSecret = process.env.ACCESS_TOKEN_SECRET;
app.post('/login', (req, res) => {
const { username, email } = req.body;
const user = { name: username, email };
const accessToken = jwt.sign(user, accessTokenSecret, { expiresIn: '1h' });
res.json({ accessToken });
});
app.get('/dashboard', authenticateToken, (req, res) => {
res.json({ message: `Welcome back, ${req.user.name}` });
});
function authenticateToken(req, res, next) {
const token = req.headers.authorization?.split(' ')[1];
jwt.verify(token, accessTokenSecret, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server running on http://localhost:${PORT}`);
});
Enhancing JWTs with User-Specific Data
Embedding additional data into JWTs can optimize both the security and efficiency of data retrieval processes, reducing the need for database queries and enhancing user experience.
Embedding Data in JWTs
- Efficiency: By embedding user-specific data such as customer ID or email, applications can reduce the frequency of database queries, which enhances performance.
- Convenience: Access to user-specific settings and features can be streamlined, improving the user experience.
- Security: Embedding specific data reduces the need to retrieve sensitive user information repeatedly, minimizing the risk of data leaks.
Practical Example:
Enhancing User Experience
app.post('/login', (req, res) => {
const { username, email } = req.body;
const user = { name: username, email };
const accessToken = jwt.sign(user, accessTokenSecret, { expiresIn: '1h' });
res.json({ accessToken });
});
Refresh Tokens Extend Authentication
Refresh tokens provide a way to maintain user sessions for extended periods without compromising security. Unlike access tokens, which are short-lived and used for a single session, refresh tokens have a longer lifespan and can be used to request new access tokens.
Why Use Refresh Tokens?
- Longevity: Refresh tokens can remain valid for days, weeks, or even months, reducing the need for users to frequently re-authenticate.
- Security: By using a refresh token to obtain a new access token, you minimize the risk associated with longer-lived access tokens. If an access token is compromised, it has a short window of validity.
Implementing Refresh Tokens
Here's an example of how you might implement refresh tokens in a NodeJS application using JWTs:
app.post('/login', (req, res) => {
const { username, email } = req.body;
const user = { name: username, email };
const accessToken = jwt.sign(user, accessTokenSecret, { expiresIn: '15m' });
const refreshToken = jwt.sign(user, refreshTokenSecret, { expiresIn: '7d' });
res.json({ accessToken, refreshToken });
});
app.post('/refresh', (req, res) => {
const { token } = req.body;
if (!token) {
return res.sendStatus(401);
}
try {
const user = jwt.verify(token, refreshTokenSecret);
const newAccessToken = jwt.sign({ name: user.name, email: user.email }, accessTokenSecret, { expiresIn: '15m' });
res.json({ accessToken: newAccessToken });
} catch (error) {
return res.sendStatus(403);
}
});
This example shows how a refresh token is generated along with an access token during the login process. When the access token expires, the refresh token can be used to generate a new one without asking the user to log in again.
Best Practices for Refresh Tokens
- Secure Storage: Always store refresh tokens securely to prevent unauthorized access.
- Revocation Strategy: Implement a strategy for revoking refresh tokens when they are no longer needed or if a user logs out. By incorporating refresh tokens into your authentication strategy, you can improve user experience by providing seamless access while maintaining high levels of security.
- Contextual Use of Refresh Tokens:
- When to Use:
- Refresh tokens are ideal for applications where user sessions need to be maintained over long periods without requiring frequent re-authentication, such as social media platforms (e.g., LinkedIn) or content streaming services. These applications benefit from the convenience of persistent sessions to enhance user experience.
- When to Avoid:
- Avoid using refresh tokens in highly secure applications, such as banking or healthcare apps, where the security risks associated with longer session durations outweigh the convenience. In these scenarios, requiring users to authenticate more frequently can prevent potential security breaches by minimizing the window of opportunity for unauthorized access if a token is compromised.
- When to Use:
Conclusion
Understanding and implementing JWTs, along with the strategic use of refresh tokens, can significantly enhance the authentication of your web applications. By ensuring that JWTs securely carry relevant user details, and by utilizing refresh tokens for maintaining longer user sessions where appropriate, developers can build more robust, efficient, and user-friendly systems. Incorporating refresh tokens offers a balance between user convenience and security, particularly in applications where users are expected to remain logged in over extended periods. Always ensure that sensitive information is handled with care to maintain privacy and security standards.
PS
-
These insights and tips on JWT implementation are derived from my diverse experiences, starting with my time at UTD's software development program, and evolving into more complex security practices with my internship with iServiceWeb.
-
Refresh token was specificlly brought to my attention by Indraneel Parthasarathy my coworker at iServiceWeb. This guy is an amazing backend developer who helped me strengthen my backend development skills.
-
If you have any questions or comments, please reach out to me on LinkedIn (don't hesitate if you see that I wrote something incorrect)