๐ A Shift In Developers Tools
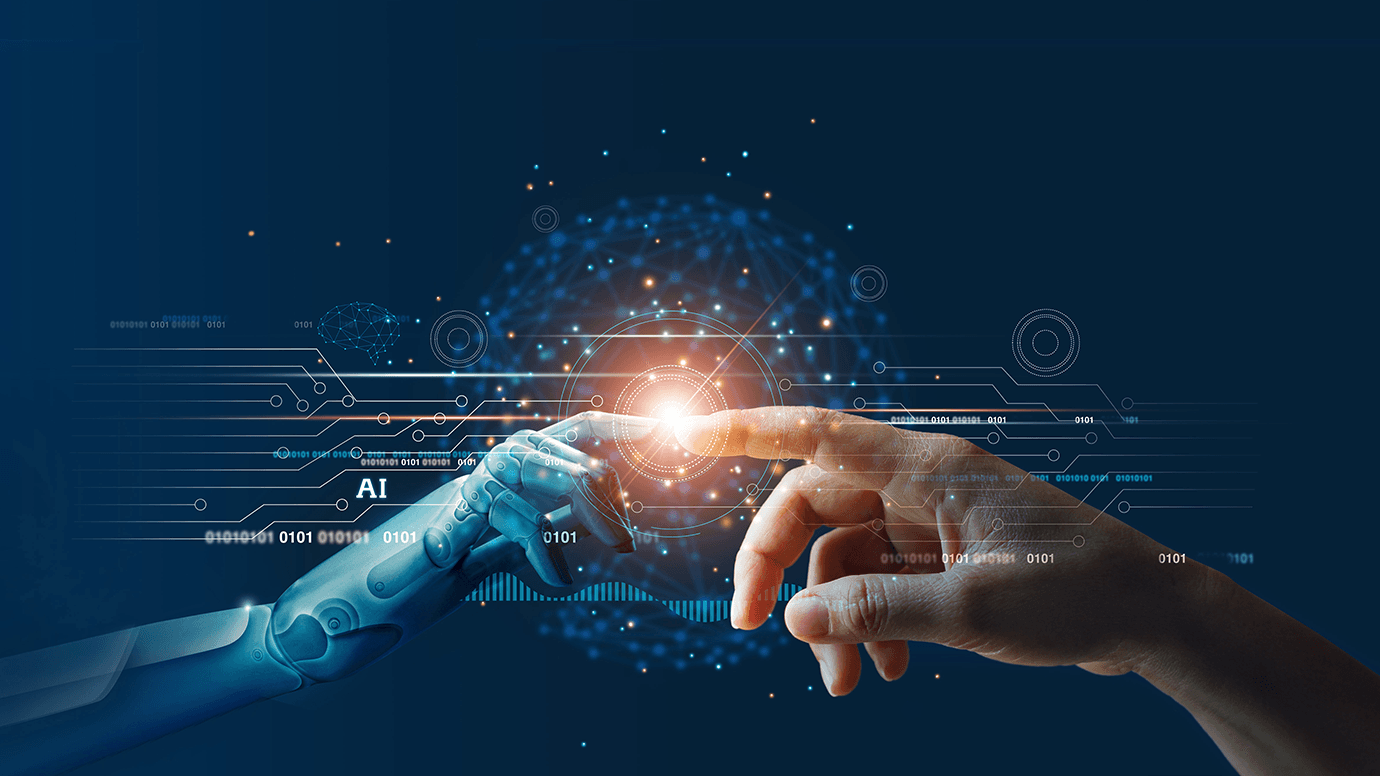
Table Of Content
- The Stack Overflow Mystery ๐ค
- **How AI Models Can Help You Become a Better Developer ๐**
- ย Tackling Coding Problems
- ย Drafting Pseudo Code First
- ย Mastering Loops and Other Concepts
- ย Understanding Confusing Code
- ย Writing Documentation
- ย Generating Test Cases
- ย Creating Boilerplate Projects
- Example folder structure prompt
- ย Generating Sample Data
- ย Debugging Issues
- Advanced AI-Integrated Tools: Cursor and Codeium
- Stack OverflowAI: A Game-Changer? ๐
- ย PS
In the world of software development, Stack Overflow has long been the go-to platform for developers seeking answers to their coding questions. It's been the treasure chest of programming knowledge, rescuing developers in times of coding trouble. However, a remarkable transformation is underwayโStack Overflow saw dip in its web traffic and it hasn't recovered sine. What was the shake up? AI or LLMs were launched allowing developers to have a coding assistant that could answer all their questions...
The Stack Overflow Mystery ๐ค
Stack Overflow, our trusted coding companion, has been the go-to platform for developers when theyโre stuck. With its massive community and structured Q&A, it's often been referred to as the holy grail for programmers. Creating a Stack Overflow account felt like earning a superhero cape for tackling coding challenges!
However, in 2023, Stack Overflow's web traffic saw a noticeable decline. This shift coincided with the rise of powerful AI tools that revolutionized how developers sought help. These tools, acting like coding sidekicks, could understand questions in plain English and provide speedy, chat-style solutions. It was a game-changing moment, and developers quickly embraced these innovations.
Despite the drop in traffic, Iโve made it a point to check Stack Overflow more often and even contribute to the community myself. Thereโs still immense value in its structured, peer-reviewed knowledge base, and contributing not only helps others but sharpens my own skills as a developer. In a world where AI is evolving rapidly, Stack Overflow remains a cornerstone for community-driven problem-solving.
How AI Models Can Help You Become a Better Developer ๐
Tackling Coding Problems
AI models like ChatGPT can help you get a better grasp of how to approach coding problems. They provide clear explanations and guidance on breaking down complex tasks into manageable steps.
- Practical Use Case: When working on HackerRank like problems, you can copy and paste the problem into an AI model and ask it to explain how to solve it in plain English. Then, use that explanation to write your code in the required programming language. This bridges the gap between understanding a problem and implementing a solution.
// Problem: Find the maximum number in an array
function findMax(nums) {
// Set max to the first number in the array
let max = nums[0];
// Loop through each number in the array
for (let num of nums) {
// If the current number is greater than max, update max
if (num > max) {
max = num;
}
}
// Return the largest number found
return max;
}
Drafting Pseudo Code First
Before writing the actual code, one way I like to use AI models is by asking them to generate pseudo code. This allows me to visualize the logical steps involved in solving the problem without getting caught up in syntax too early.
- Prompt: "Give me pseudo code for checking if a string is a palindrome"
// Example AI-generated pseudo code:
function isPalindrome(str){
// convert str to lowercase
// remove non-alphanumeric characters
// reverse the string
// compare with original
// return true if equal, else false
}
- Why This Helps: Drafting pseudo code builds a roadmap in your mind, making the actual implementation feel more like translating rather than inventing from scratch. Itโs also a great skill to develop for technical interviews and collaborative planning.
// Final implementation based on pseudo code:
function isPalindrome(str) {
// Convert the string to lowercase
const cleaned = str.toLowerCase()
// Remove all non-alphanumeric characters
.replace(/[^a-z0-9]/g, '');
// Reverse the cleaned string
const reversed = cleaned.split('').reverse().join('');
// Compare the cleaned string with the reversed version
return cleaned === reversed;
}
Mastering Loops and Other Concepts
Like many developers, loops were tricky to me at first. AI models provide practical advice, examples, and insights into effectively using for
and while
loops.
-
Prompt: "When should I use a
for
loop or awhile
loop in JavaScript?" -
AI Explanation:
- Use a
for
loop when you know how many times you want to iterate (e.g. looping over arrays, running a task a specific number of times). - Use a
while
loop when the number of iterations is unknown or based on a condition that might change during runtime.
- Use a
// FOR LOOP
// Best when you know how many times you want to loop
for (let i = 0; i < 5; i++) {
console.log(i); // Prints 0 through 4
}
// WHILE LOOP
// Best when looping depends on a dynamic condition
let count = 0;
while (count < 5) {
console.log(count); // Prints 0 through 4
count++;
}
Understanding Confusing Code
Sometimes, you inherit a codebase or encounter code written by someone else thatโs hard to understand. AI tools can make this process much easier.
- How It Works: Copy and paste the confusing code into ChatGPT and ask for an explanation. AI can break down the code line by line, explaining what each part does.
- Why Itโs Helpful: This saves time, boosts your confidence, and ensures that you understand the functionality before making changes.
// Example confusing code
const result = arr.reduce((acc, val) => acc + val, 0);
// AI breakdown:
// 1. Starts with acc = 0
// 2. Adds each value in arr to acc
// 3. Returns the total sum
Writing Documentation
AI models excel at generating clear, concise, and professional documentation. Whether itโs API documentation, project guides, or overviews, they save time and ensure effective communication.
/**
* Adds two numbers
* @param {number} a - First number
* @param {number} b - Second number
* @returns {number} Sum of a and b
*/
function add(a, b) {
return a + b;
}
Generating Test Cases
AI models can assist in creating test cases for your code, including unit tests and integration tests.
// Example test case
describe('add', () => {
it('should return the sum of two numbers', () => {
expect(add(2, 3)).toBe(5);
});
});
Creating Boilerplate Projects
AI tools can kickstart a project by generating initial structures or basic outlines.
# Example folder structure prompt
my-app/
โโโ public/
โโโ src/
โ โโโ components/
โ โโโ pages/
โ โโโ App.jsx
โโโ tailwind.config.js
โโโ package.json
โโโ index.html
Generating Sample Data
For front-end developers waiting on backend APIs, AI models are a lifesaver. They can generate mock data in JSON or other formats for testing.
[
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"phone": "123-456-7890"
},
{
"id": 2,
"name": "Jane Smith",
"email": "jane@example.com",
"phone": "098-765-4321"
}
]
Debugging Issues
Stuck with a bug? Paste the error message or problematic code into ChatGPT and ask something like:
- Prompt: "Why am I getting this error in my JavaScript function?"
AI tools can offer a fresh perspective, sometimes catching small issues like off-by-one errors or incorrect syntax.
// Buggy code:
const arr = [1, 2, 3];
console.log(arr(0)); // โ TypeError: arr is not a function
// AI Suggests:
// Use square brackets [] to access array elements
console.log(arr[0]); // โ
1
- Why This Helps: Having a second set of eyesโeven if it's an AIโcan help you break through frustrating bugs faster and reinforce best practices.
Advanced AI-Integrated Tools: Cursor and Codeium
But that's not all. Developers are not just embracing AI tools for quick code snippets, concept explanations, or debugging help. Tools like Cursor and Codeium are also revolutionizing the way we code. Here's a quick look:
-
Cursor AI Editor: Cursor is an innovative code editor with built-in AI features, such as AI chat and autocompletion. Its seamless integration of AI makes coding more efficient. While it's still evolving and has some stability issues on certain platforms, itโs paving the way for AI-assisted development environments.
-
Codeium: A powerful alternative to Cursor, Codeium offers AI-driven code completion, free AI chat support, and compatibility with a wide range of IDEs, including VS Code. Itโs known for its speed and accuracy, making it a reliable choice for enhancing productivity.
Stack OverflowAI: A Game-Changer? ๐
The introduction of AI-driven tools like ChatGPT, Gemini, MetaAI, Claude Cursor, and Codeium has provided lightning-fast solutions. With IDE extensions in the mix, theyโre making coding even more fun and efficient. As developers jump on the AI and community-driven bandwagon, theyโre encouraged to stay sharp and use these resources to turbocharge their coding adventures. The future promises a dynamic world where AI and community wisdom live harmoniously. Happy coding! ๐ฎ๐
PS
- If you have any questions or comments, please reach out to me on LinkedIn (don't hesitate if you see something wrong).